✨ JavaScript Interview Questions And Answers | Codemate ✨
“Today, we’re diving into the world of Codemate , a company renowned for its commitment to technical excellence and effective communication. As we explore a series of JavaScript interview questions, we’ll see how Codemate values of clarity, efficiency, and logical thinking manifest in the solutions candidates provide. Join us as we delve into these coding challenges and unravel the essence of what makes Codemate approach to talent assessment unique and effective.”
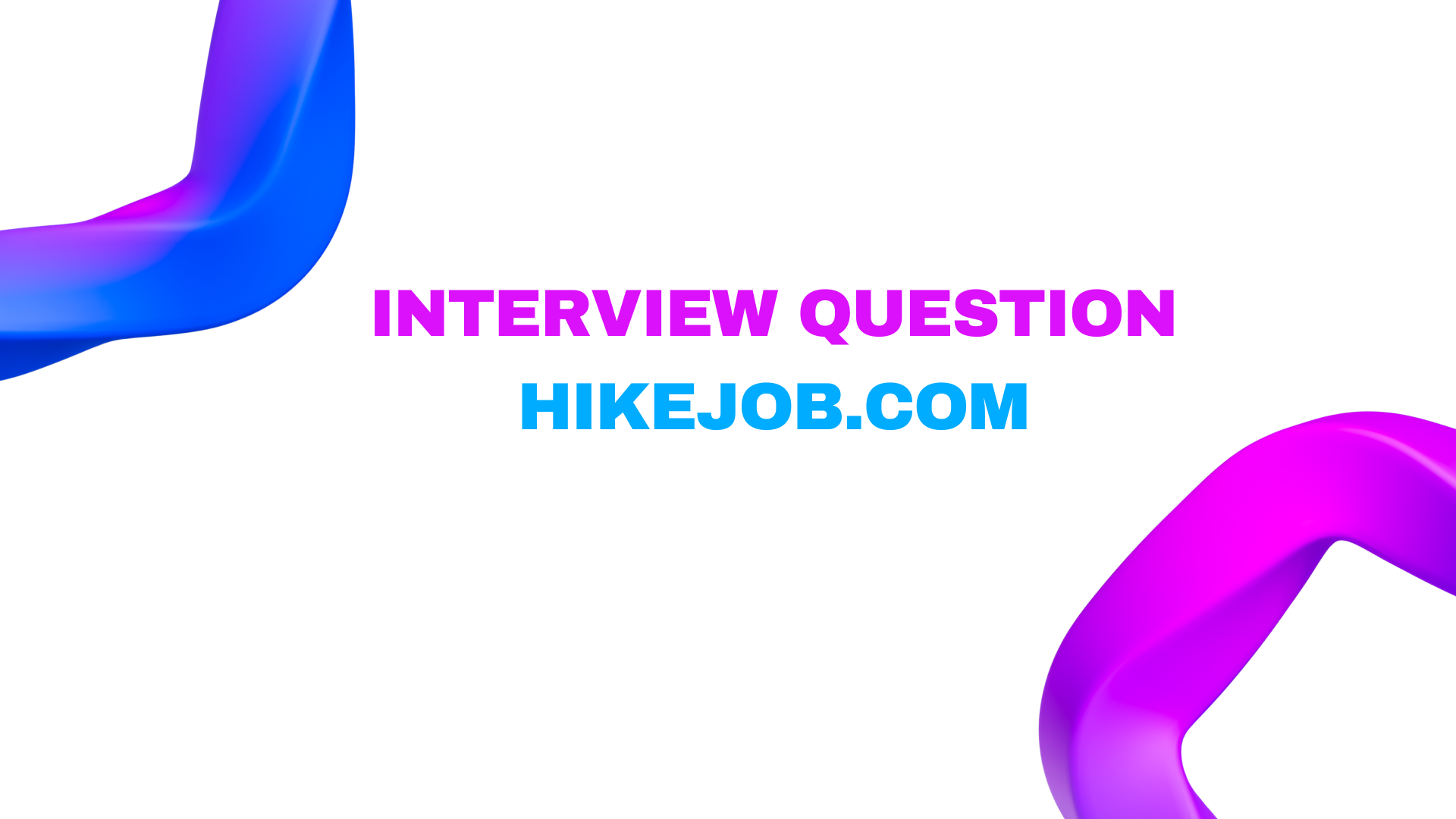
Question: Write a Function to calculate the sum of two numbers.
When managers pose this question, they aim to gauge candidates’ fundamental grasp of JavaScript, including basic syntax and problem-solving abilities. It’s also an opportunity to assess their coding approach and attention to detail.
For instance, a candidate might respond by presenting a simple function designed to calculate the sum of two numbers provided as arguments:
function calculateSumOfTwo(x, y){
return x+y;
}
Question: Write a program to find the maximum number in an array.
When posed with this question, hiring managers seek insight into candidates’ capacity to craft concise and effective code. It’s pivotal for candidates to elucidate their code methodically, showcasing code that is both error-free and efficient.
For instance, a candidate might respond with the following function aimed at finding the maximum number in an array:
function findMaxNumber(inputArray) {
return Math.max(…inputArray);
}
In this function named findMaxNumber
, the input parameter inputArray
represents the array within which the maximum number is to be found. By employing the Math.max() function along with the spread operator (…), the function efficiently returns the maximum value from the array. This demonstration not only exhibits the candidate’s command over JavaScript but also their ability to write clear and succinct code.
Question: Write a program to find the maximum number in an array.
The interviewer aims to assess candidates’ proficiency in loop constructs, JavaScript string methods, and other fundamental JavaScript syntax through their approach to solving the palindrome problem.
For instance, a candidate might address this by presenting a function like so:
function checkPalindrome(inputString) {
const reversedString = inputString.split('').reverse().join('');
return inputString === reversedString;
}
In this function named checkPalindrome
, the input parameter inputString
represents the string being evaluated. By splitting the string into an array of characters, then reversing that array, and finally joining it back into a string, we create reversedString
. The function then compares inputString
with reversedString
to determine if it’s a palindrome. This showcases the candidate’s familiarity with JavaScript’s string manipulation methods and their ability to solve problems effectively.
Question: Write a program to reverse a given string.
Hiring managers anticipate precise solutions that exhibit the interviewee’s adeptness in JavaScript programming.
For instance, a candidate might offer the following function:
const reverseString = (inputStr) => {
return inputStr.split('').reverse().join('');
};
In this example, the function reverseString
takes an input string inputStr
, splits it into an array of characters, reverses the order of the characters, and then joins them back into a string. This succinct yet accurate solution underscores the candidate’s proficiency in JavaScript.
Question: Write a function that takes an array of numbers and returns a new array with only the even numbers
Interviewers seek candidates capable of articulating their logic clearly, alongside providing code solutions, showcasing logical thinking processes.
For instance, to filter even numbers from an array, one might employ the filter
method, checking each element’s evenness using the modulus operator (%), where a remainder of 0 indicates evenness. Here’s a representation:
function filterEvenNumbers(inputNumbers) {
return inputNumbers.filter(number => number % 2 === 0);
}
In this code, the filterEvenNumbers
function takes an array inputNumbers
, then employs the filter
method to create a new array containing only the elements that pass the condition of being even. This solution not only provides the code but also explains the approach, demonstrating the candidate’s logical thinking and ability to articulate their reasoning.